⯈Algorithm and its Characteristics
⯈Elementary Problems
- Addition of two numbers
- Calculate area and circumference of circle
- Calculate area of triangle
- Calculate simple interest
- Calculate slope and distance between two points
- Convert length in feet to inches
- Weighted score in exam
- Convert temperature in degree Celsius to Fahrenheit
- Swap two numbers
- Swap two numbers without using extra variable
- Overview of C Programming Language
- Getting started with C Programming Language
- Keywords
- Identifiers
- Constants
- Operators
- Expression Evaluation
- Mathematical expression to C equivalent expressions
- Datatypes
- Variables
- Integer representation in C
- Character representation in C
- Type conversion in C
- sizeof operator
- Comments
- Mathematical Functions
- input output statements
- width specifiers in C
- structure of a C program
- header files
- Compilation process of a C program
- Types of initializations.
⯈Basic C Programs
- C program to add two numbers
- C program to find area and circumference of circle
- C program to swap two numbers
- C program to swap two numbers without using extra variable
- C program to swap two numbers using bitwise XOR
- C program to convert temperature in Celsius to Fahrenheit
- C program to calculate gross salary of an employee
- C program to count number of digits in a positive integer
- C program to count number of digits in binary representation
- C program to count number of digits in base ‘K’
- C program to convert kilometer to meter, feet, inches and centimeters
- C program to find first and last digit of a number
- C program to find minimum number of currency denominations
- C program to convert cartesian coordinates to polar coordinates
- C program to find distance between two places on earth in nautical miles
- C program to find slope and distance between two points
- C program to add 1 to the number using ‘+’ operator
- C program to find maximum of two numbers using ternary operator
- C program to find maximum of three numbers using ternary operator
- C program to find kth bit of a number
- C program to find last four bits of a byte
- C Program to reset right most set bit of a number
Structure of a C Program
The structure of a C program consists of the following:
- Documentation Section.
- Pre-processor directives.
- Definition section.
- Global declaration.
- main function.
- sub-program section.
We shall discuss these in detail.
You may watch the video posted at the end of this page.
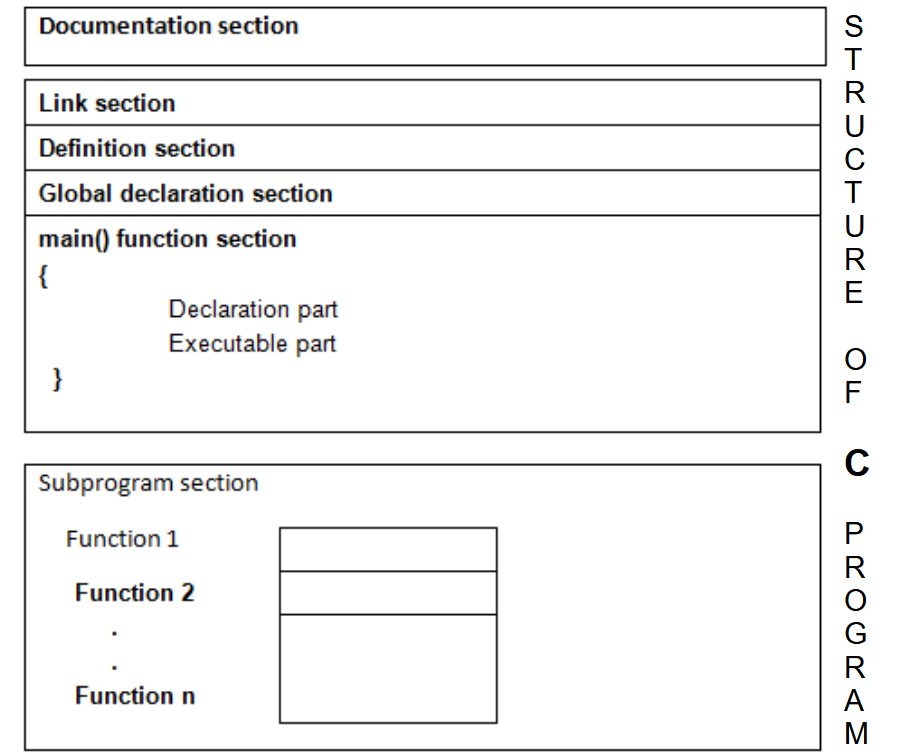
Documentation Section
- In this section, we may write.
- Name of program.
- Author Name.
- Date on which program was written.
- Tester name.
- Version of the code.
- Inputs and Outputs.
All these are written in the form of comments.
/*
Name of Program:
Author Name:
Date:
Input:
Output:
Tester Name:
Version Name:
etc.,
*/
pre-processor directives
In this section we write all the header files. In header files there will be multiple functions defined (may be built-in or user-defined), which helps in reusability of the code.
We include header files as:
#include<stdio.h>
#include<stdlib.h>
Definition section
In this section we write MACROS and mainfest constants. These will get replaced through-out the program by the pre-processor. The pre-processor begins with ‘#’, and whenever we write something that begins with ‘#’ we don’t put semicolon at the end of the statement.
Let us see some examples,
#define PI 3.142
- Wherever we write PI, the pre-processor will replace it with 3.142 during the 1st pass of the compiler.
- Normally mainfest constants are written in upper-case. (A standard, not mandatory)
Global declaration section
In this section we write,
- Global variables
- Function declaration
- Static variables
Variables or functions declared in this section can be used anywhere in the program.
main function
- main function is the starting point of any program, unless or otherwise specified or changed.
- main is a user-defined function.
- The return type of the main program is int.
int main()
{
local declaration
......
return 0;
}
sub-program section
- These are the user-defined functions that we write here.
- There can be n number of sub-programs.
- Each sub-program performs the particular task. (unique task)
- Sub-programs are written for code reusability purpose.