⯈Algorithm and its Characteristics
⯈Elementary Problems
- Addition of two numbers
- Calculate area and circumference of circle
- Calculate area of triangle
- Calculate simple interest
- Calculate slope and distance between two points
- Convert length in feet to inches
- Weighted score in exam
- Convert temperature in degree Celsius to Fahrenheit
- Swap two numbers
- Swap two numbers without using extra variable
- Overview of C Programming Language
- Getting started with C Programming Language
- Keywords
- Identifiers
- Constants
- Operators
- Expression Evaluation
- Mathematical expression to C equivalent expressions
- Datatypes
- Variables
- Integer representation in C
- Character representation in C
- Type conversion in C
- sizeof operator
- Comments
- Mathematical Functions
- input output statements
- width specifiers in C
- structure of a C program
- header files
- Compilation process of a C program
- Types of initializations.
⯈Basic C Programs
- C program to add two numbers
- C program to find area and circumference of circle
- C program to swap two numbers
- C program to swap two numbers without using extra variable
- C program to swap two numbers using bitwise XOR
- C program to convert temperature in Celsius to Fahrenheit
- C program to calculate gross salary of an employee
- C program to count number of digits in a positive integer
- C program to count number of digits in binary representation
- C program to count number of digits in base ‘K’
- C program to convert kilometer to meter, feet, inches and centimeters
- C program to find first and last digit of a number
- C program to find minimum number of currency denominations
- C program to convert cartesian coordinates to polar coordinates
- C program to find distance between two places on earth in nautical miles
- C program to find slope and distance between two points
- C program to add 1 to the number using ‘+’ operator
- C program to find maximum of two numbers using ternary operator
- C program to find maximum of three numbers using ternary operator
- C program to find kth bit of a number
- C program to find last four bits of a byte
Algorithm and Flowchart to add two numbers
Whenever we write an algorithm or program, “always read the input first”. The input to this algorithm is any two numbers. So let us assume that A and B be some numbers. To read the input from the end user, we use “Read“, some use “Input“, that is perfectly ok to use anyone.
So, two read the input we may write,
Read two numbers as A and B. you could even write as Read A and B. The former is more appealing as it says A and B are numbers, so let us stick to that.
After we read two numbers, the task is to add the two numbers, so let’s add, A + B. The value of A + B should be stored in some variable. A variable is like a container that holds some value. (More about variables we will discuss in the Basics of C Programming chapter.) Hence, we store the answer in a variable. Let the variable name be Sum.
Therefore, we write,
Sum = A + B, read as Sum is assigned with the value of A + B.
At the end the algorithm should have at least one output. Therefore we display the answer and that answer is stored in variable Sum. To output we use word “Display“, some use the word “Print“
We write, Display Sum.
Note: An algorithm should be generic, it shouldn’t be specific. We shouldn’t write as , “Read two numbers 3 and 4”
Algorithm:
Name of Algorithm: To add two numbers.
Step 1: Start
Step 2: Read two numbers as A and B
Step 3: Sum = A + B
Step 4: Display Sum
Step 5: Stop
Flowchart:
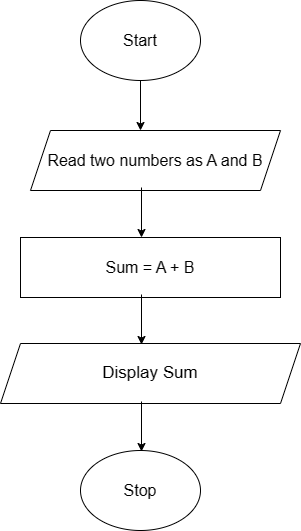
Tracing:
Let us do the dry run of the above algorithm:
At step number 2, it reads two numbers as A and B.
Let A = 10 and B = 20.
Step 3 computes, Sum = A + B, Sum = 10 + 20, Sum = 30
At Step 4: Display the value stored in Sum, i.e., 30 and we stop the algorithm.