⯈Algorithm and its Characteristics
⯈Elementary Problems
- Addition of two numbers
- Calculate area and circumference of circle
- Calculate area of triangle
- Calculate simple interest
- Calculate slope and distance between two points
- Convert length in feet to inches
- Weighted score in exam
- Convert temperature in degree Celsius to Fahrenheit
- Swap two numbers
- Swap two numbers without using extra variable
- Overview of C Programming Language
- Getting started with C Programming Language
- Keywords
- Identifiers
- Constants
- Operators
- Expression Evaluation
- Mathematical expression to C equivalent expressions
- Datatypes
- Variables
- Integer representation in C
- Character representation in C
- Type conversion in C
- sizeof operator
- Comments
- Mathematical Functions
- input output statements
- width specifiers in C
- structure of a C program
- header files
- Compilation process of a C program
- Types of initializations.
⯈Basic C Programs
- C program to add two numbers
- C program to find area and circumference of circle
- C program to swap two numbers
- C program to swap two numbers without using extra variable
- C program to swap two numbers using bitwise XOR
- C program to convert temperature in Celsius to Fahrenheit
- C program to calculate gross salary of an employee
- C program to count number of digits in a positive integer
- C program to count number of digits in binary representation
- C program to count number of digits in base ‘K’
- C program to convert kilometer to meter, feet, inches and centimeters
- C program to find first and last digit of a number
- C program to find minimum number of currency denominations
- C program to convert cartesian coordinates to polar coordinates
- C program to find distance between two places on earth in nautical miles
- C program to find slope and distance between two points
- C program to add 1 to the number using ‘+’ operator
- C program to find maximum of two numbers using ternary operator
- C program to find maximum of three numbers using ternary operator
- C program to find kth bit of a number
- C program to find last four bits of a byte
Shift operators in C
Before we discuss the shift operators, it is important for us to know how integer numbers are represented in computer. The data is stored in computers in binary language. The shift operators are bitwise operators, and they are applied on the bit pattern.
Please refer this on how we convert from one base system to another before going into the shift operators.
There are two types of shift operators
Note: Shift operators can only be applied on the integers.
You may refer to the video posted at the end of this page.
Left shift operator (<<)
The left shift operator (<<) shifts the bit pattern of a number, towards the left by specified number of bits.
It is written as, y = x<<k, means shift the bit pattern of x by k bits on the LHS and store the answer in y.
Let us understand this with the help of an example.
y = 13 << 1, read as shift the bit patten of 13 towards left by 1 bit.
We convert 13 in binary which is 1101. We write 1101 in 32 bits and shift the bit pattern towards left.
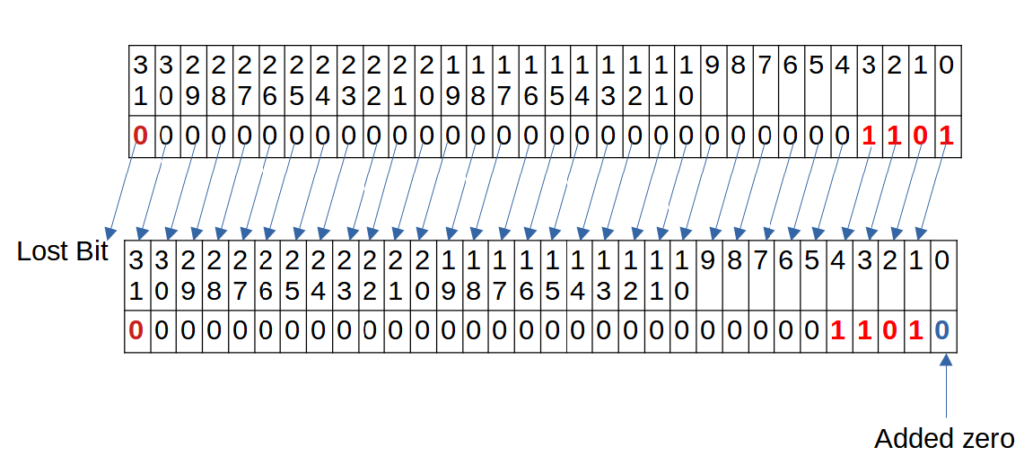
We see that the bit pattern of 13, is shifted 1 bit towards left. The MSB (31st bit) is lost.
30th bit goes to 31st bit position.
29th bit goes to 30th bit position.
28th bit goes to 29th bit position. and so on.
The 0th bit will go to 1st bit position and the 0th bit is added with 0. (Since it is empty now)
Converting the number back to decimal we get 26.
Therefore, 13<<1 = 26
Similarly, if we do 13<<2, we will get 52. (I hope that you will try it 🙂
we observe,
13<<1 = 26 =13 * 21
13<<2 = 52 =13 * 22
Therefore, in general
y = x<<k
y =x * 2k
Right shift operator (>>)
The right shift operator (>>) shifts the bit pattern of a number, towards the right by specified number of bits.
It is written as, y = x>>k, means shift the bit pattern of x by k bits on the RHS and store the answer in y.
Let us understand this with the help of an example.
y = 13 >> 1, read as shift the bit patten of 13 towards right by 1 bit.
We convert 13 in binary which is 1101. We write 1101 in 32 bits and shift the bit pattern towards right.
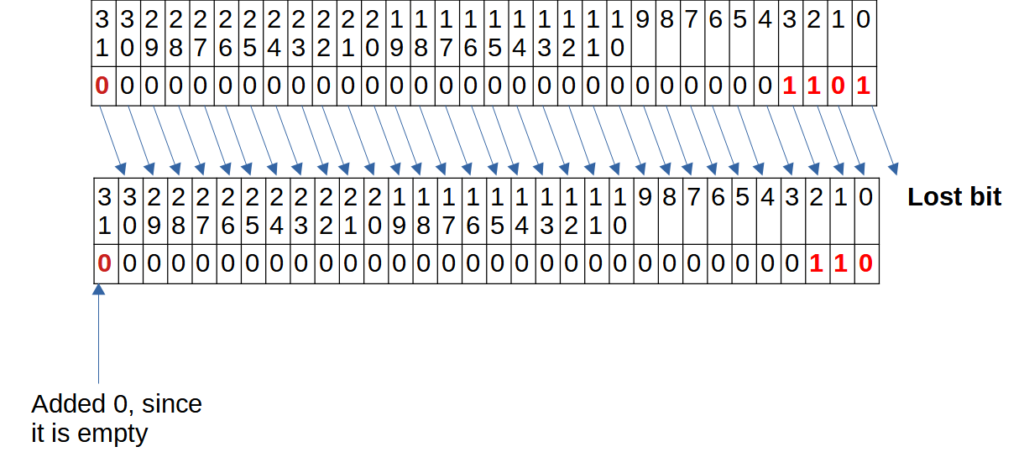
We see that the bit pattern of 13, is shifted 1 bit towards right. The LSB (0th bit) is lost.
31st bit goes to 30th bit position.
30th bit goes to 29th bit position.
29th bit goes to 28th bit position and so on.
The 1st bit will go to 0th bit position and the 0th bit is lost.
Since the MSB (31st bit) is empty we add 0.
Converting the number back to decimal we get 6.
Therefore, 13>>1 = 26
we observe,
13>>1 = 6 =13 / 21
13>>2 = 3 =13 / 22
Therefore, in general
y = x>>k
y =x / 2k
int / int = int.
Note:
- The left shift and right shift operators should not be shifted by negative number of bits. The result is undefined.
- y = x<<k
- y = x>>k
In both of the above cases the value of k>=0, if k<0, the result is undefined.
Ex:
The following results are undefined.
2<<-2 and 2>>-1
2. If the number is shifted more than the number of bits, since shift can only be applied on integer (32 bits), if we shift a number for more than 32 bits, say 1<<34, the result is undefined.
Few Examples
- 2<<3 = 8 (2 * 2³)
- 1<<k = 2k
- 1>>k = 1/2k
- 3>>2 = 0 (3/2²)
- -1<<2 = -4 (-1 * 2²)