⯈Algorithm and its Characteristics
⯈Elementary Problems
- Addition of two numbers
- Calculate area and circumference of circle
- Calculate area of triangle
- Calculate simple interest
- Calculate slope and distance between two points
- Convert length in feet to inches
- Weighted score in exam
- Convert temperature in degree Celsius to Fahrenheit
- Swap two numbers
- Swap two numbers without using extra variable
- Overview of C Programming Language
- Getting started with C Programming Language
- Keywords
- Identifiers
- Constants
- Operators
- Expression Evaluation
- Mathematical expression to C equivalent expressions
- Datatypes
- Variables
- Integer representation in C
- Character representation in C
- Type conversion in C
- sizeof operator
- Comments
- Mathematical Functions
- input output statements
- width specifiers in C
- structure of a C program
- header files
- Compilation process of a C program
- Types of initializations.
⯈Basic C Programs
- C program to add two numbers
- C program to find area and circumference of circle
- C program to swap two numbers
- C program to swap two numbers without using extra variable
- C program to swap two numbers using bitwise XOR
- C program to convert temperature in Celsius to Fahrenheit
- C program to calculate gross salary of an employee
- C program to count number of digits in a positive integer
- C program to count number of digits in binary representation
- C program to count number of digits in base ‘K’
- C program to convert kilometer to meter, feet, inches and centimeters
- C program to find first and last digit of a number
- C program to find minimum number of currency denominations
- C program to convert cartesian coordinates to polar coordinates
- C program to find distance between two places on earth in nautical miles
- C program to find slope and distance between two points
- C program to add 1 to the number using ‘+’ operator
- C program to find maximum of two numbers using ternary operator
- C program to find maximum of three numbers using ternary operator
- C program to find kth bit of a number
- C program to find last four bits of a byte
- C Program to reset right most set bit of a number
Algorithm and Flowchart to calculate area and circumference of circle
Whenever we write an algorithm or program, we always start with reading the input. The input to this algorithm is radius (R) of a circle.
Formula:
area of circle = ΠR2 ,
circumference of circle = 2ΠR.
Note:
- The value of Pi is constant, and constants should not be read as an input.
- Read only the values of variables that are part of input.
So, lets read the radius, hence we write Read radius as R
After reading all the necessary inputs it’s the time to calculate the area and circumference.
a = ΠR2
c = 2ΠR
Then we need to output the results. The results are stored in variables a and c.
Note: Variables names are case – sensitive.
Therefore, we write
Display a
Display c
Algorithm:
Name of Algorithm: Compute area and circumference of circle.
Step 1: Start
Step 2: Read radius as R.
Step 3: a = ΠR2
Step 5: Display a [outputs the content stored in variable ‘a’]
Step 6: Display c [outputs the content stored in variable ‘c’]
Sep 7: Stop
Note: We can use mathematical symbols in an algorithm, but cannot be used in programs.
Flowchart:
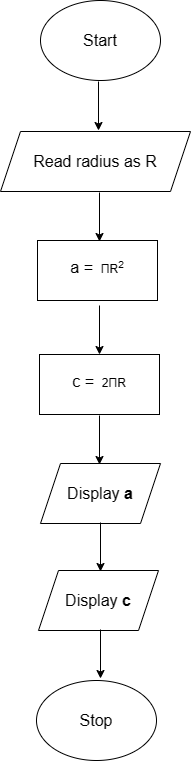
Tracing:
Let us do the dry run of the above algorithm:
In step 2, we read the value of radius (R). Let R = 1.5
In step 3 and step 4 we calculate area and circumference and store the result in ‘a’ and ‘c’ respectively.
a =22/7 * 1.52 = 7.071
c = 2 * 22/7 * 1.5 = 9.428
In step 5, and 6, we display the value stored in variable a and c. Therefore we output 7.071 and 9.428