⯈Algorithm and its Characteristics
⯈Elementary Problems
- Addition of two numbers
- Calculate area and circumference of circle
- Calculate area of triangle
- Calculate simple interest
- Calculate slope and distance between two points
- Convert length in feet to inches
- Weighted score in exam
- Convert temperature in degree Celsius to Fahrenheit
- Swap two numbers
- Swap two numbers without using extra variable
- Overview of C Programming Language
- Getting started with C Programming Language
- Keywords
- Identifiers
- Constants
- Operators
- Expression Evaluation
- Mathematical expression to C equivalent expressions
- Datatypes
- Variables
- Integer representation in C
- Character representation in C
- Type conversion in C
- sizeof operator
- Comments
- Mathematical Functions
- input output statements
- width specifiers in C
- structure of a C program
- header files
- Compilation process of a C program
- Types of initializations.
⯈Basic C Programs
- C program to add two numbers
- C program to find area and circumference of circle
- C program to swap two numbers
- C program to swap two numbers without using extra variable
- C program to swap two numbers using bitwise XOR
- C program to convert temperature in Celsius to Fahrenheit
- C program to calculate gross salary of an employee
- C program to count number of digits in a positive integer
- C program to count number of digits in binary representation
- C program to count number of digits in base ‘K’
- C program to convert kilometer to meter, feet, inches and centimeters
- C program to find first and last digit of a number
- C program to find minimum number of currency denominations
- C program to convert cartesian coordinates to polar coordinates
- C program to find distance between two places on earth in nautical miles
- C program to find slope and distance between two points
- C program to add 1 to the number using ‘+’ operator
- C program to find maximum of two numbers using ternary operator
- C program to find maximum of three numbers using ternary operator
- C program to find kth bit of a number
- C program to find last four bits of a byte
- C Program to reset right most set bit of a number
Algorithm and Flowchart to swap two numbers
Swapping, exchange the values of two variables. The swapping is used in many algorithms, to name we use swapping in selection sort, bubble sort, etc.
The input to this algorithm is two numbers. Let the two numbers be A and B. After swapping is performed, the contents of A and B has to be exchanged.
Ex:
Let A = 10 and B = 20
After swapping,
A = 20 and B = 10
There are two methods with which we can swap. (Actually three, the last one is using bitwise XOR, which we will discuss in next chapter)
Method 1: Using temporary variable.
Let, A = 10 and B = 20
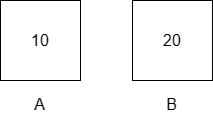
If we write A = B and B = A, this will produce the wrong answer. Because the contents of A will be overwritten with B, and the value of A is lost.
Check the diagram below for more detailed explanation.
Note: A variable can only store one value at a given point of time, if we store the new value the previous value will be overwritten, variables say "Chhodo kal Ki Baatein"
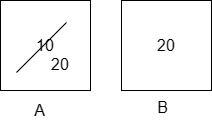
The value of A becomes 20, and the value 10 is lost. Therefore, if we write B = A now, B will also be 20.
It means the value of A is formatted by the statement A = B. Before we format, we take the back up of A, so that we can use the value of A later to store in B.
Therefore, we write,
temp = A [taking back-up of variable A]

Now we can overwrite the value of A, since the backup is taken.
A = B
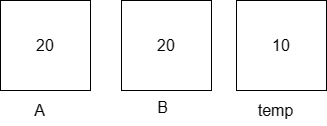
At last, we can clearly see that B should get the value of temp.
B = temp
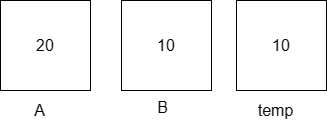
At end we see that the values of A and B are swapped.
Algorithm:
Name of Algorithm: To swap two numbers.
Step 1: Start
Step 2: Read two numbers as A and B.
Step 3: temp = A
A = B
B = temp
Step 4: Display A and B
Step 5: Stop
Flowchart:
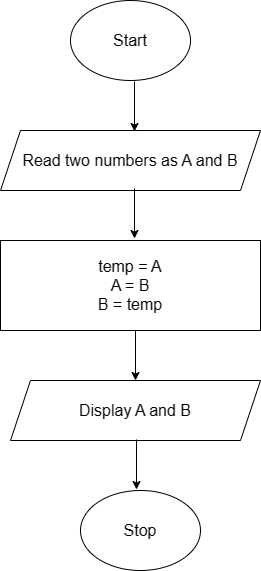
In the next section we will see how to swap two integers without using extra variable.