⯈Algorithm and its Characteristics
⯈Elementary Problems
- Addition of two numbers
- Calculate area and circumference of circle
- Calculate area of triangle
- Calculate simple interest
- Calculate slope and distance between two points
- Convert length in feet to inches
- Weighted score in exam
- Convert temperature in degree Celsius to Fahrenheit
- Swap two numbers
- Swap two numbers without using extra variable
- Overview of C Programming Language
- Getting started with C Programming Language
- Keywords
- Identifiers
- Constants
- Operators
- Expression Evaluation
- Mathematical expression to C equivalent expressions
- Datatypes
- Variables
- Integer representation in C
- Character representation in C
- Type conversion in C
- sizeof operator
- Comments
- Mathematical Functions
- input output statements
- width specifiers in C
- structure of a C program
- header files
- Compilation process of a C program
- Types of initializations.
⯈Basic C Programs
- C program to add two numbers
- C program to find area and circumference of circle
- C program to swap two numbers
- C program to swap two numbers without using extra variable
- C program to swap two numbers using bitwise XOR
- C program to convert temperature in Celsius to Fahrenheit
- C program to calculate gross salary of an employee
- C program to count number of digits in a positive integer
- C program to count number of digits in binary representation
- C program to count number of digits in base ‘K’
- C program to convert kilometer to meter, feet, inches and centimeters
- C program to find first and last digit of a number
- C program to find minimum number of currency denominations
- C program to convert cartesian coordinates to polar coordinates
- C program to find distance between two places on earth in nautical miles
- C program to find slope and distance between two points
- C program to add 1 to the number using ‘+’ operator
- C program to find maximum of two numbers using ternary operator
- C program to find maximum of three numbers using ternary operator
- C program to find kth bit of a number
- C program to find last four bits of a byte
- C Program to reset right most set bit of a number
C Program to add two numbers
Whenever we start the program always, we start with reading the inputs and calculating the outputs.
Input: Two numbers. (int)
Output: Sum of two numbers. (int)
We read the inputs using runtime initialization, so we write the scanf statement as,
scanf("%d%d",&a,&b);
Let ‘a’ and ‘b’ be the two integers as input.
No variables can be used unless they are declared.
Hence, we update the program as,
int main()
{
int a, b;
scanf("%d%d",&a,&b);
}
We calculate the output after reading all the necessary inputs,
sum = a+b
Hence, the updated program will be,
Note:
1. No variable should be used unless it is declared.
2. The value of the variable on RHS of ‘=’, its value should be known before its first point of usage.
int main()
{
int a, b;
int sum;
scanf("%d%d",&a,&b);
sum = a + b;
}
An algorithm should have at least one output, the answer is stored in variable sum. Hence, we need to print it.
printf("sum = %d\n",sum);
The final program becomes,
Since, we are using printf and scanf, we need to include stdio.h as these files are present in these header files.
#include<stdio.h>
int main()
{
int a, b;
int sum;
scanf("%d%d",&a,&b);
sum = a + b;
printf("sum = %d\n",sum);
return 0;
}
Output window of above program
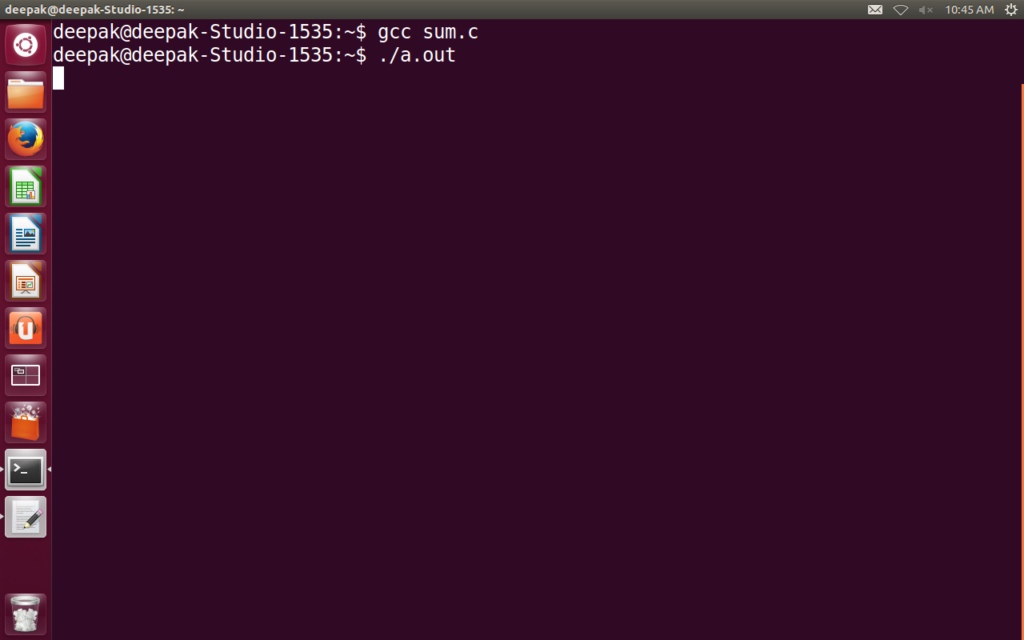
Let the two numbers be 3 and 4, We see that we get the output.
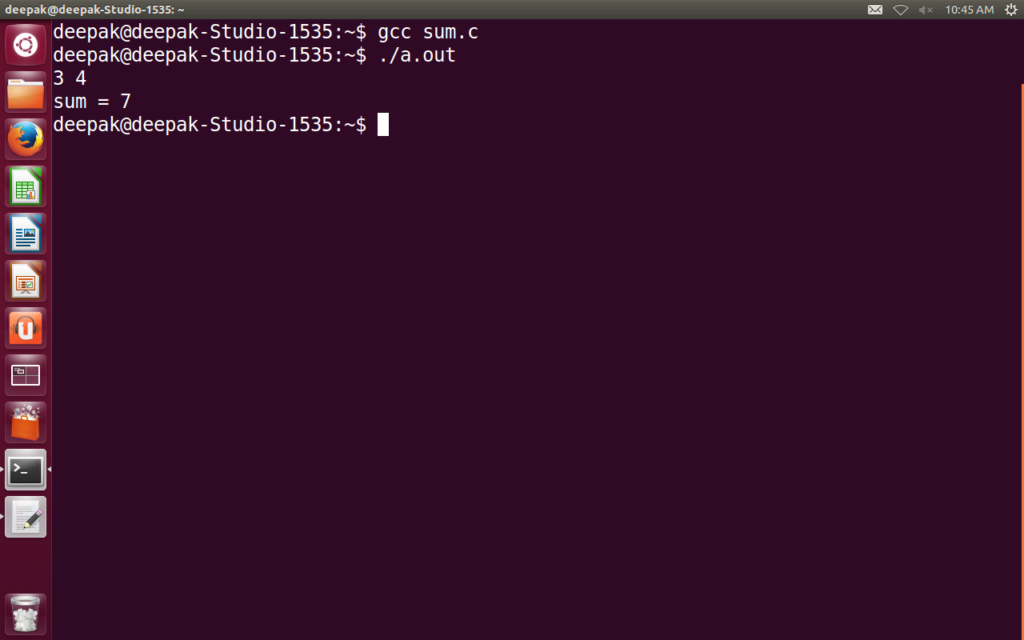
We see that the cursor is blinking, and we don’t know what to enter. Shall we enter number or a string, its not clear.
Its like the form given below to fill the details, but the captions are not provided.
In order to have good user interface, we can provide a message before reading the numbers as, (Optional, user interface may or may not be provided)
#include<stdio.h>
int main()
{
int a, b;
int sum;
printf("Enter two numbers:");
scanf("%d%d",&a,&b);
sum = a + b;
printf("sum = %d\n",sum);
return 0;
}
Output window of above program
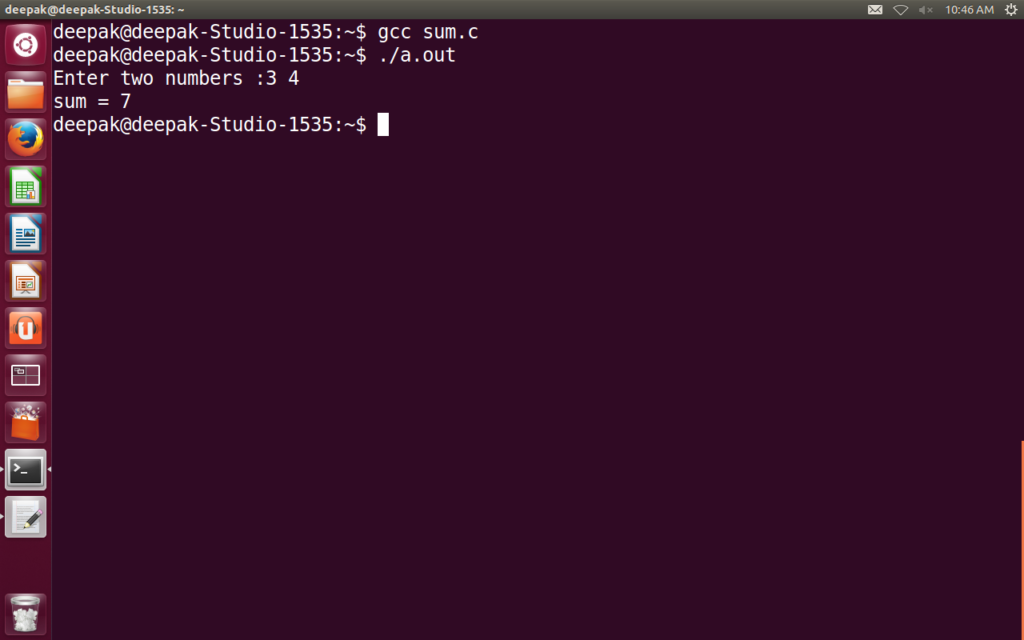
We see that there is a user interface asking for Enter two numbers: which gives more meaning for end user, what to give as an input.
Memory layout of above program
The scope after declaring all the variables,

Scope after reading the values of ‘a’ and ‘b’.
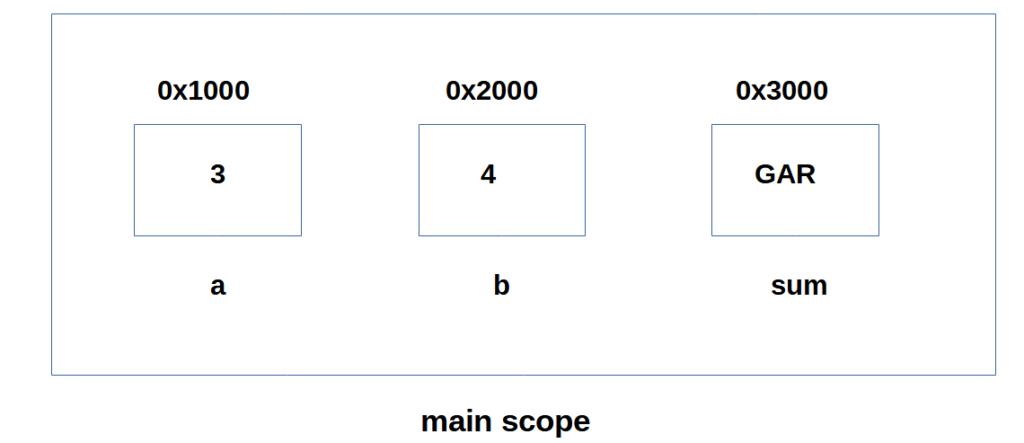
Scope after calculating the sum
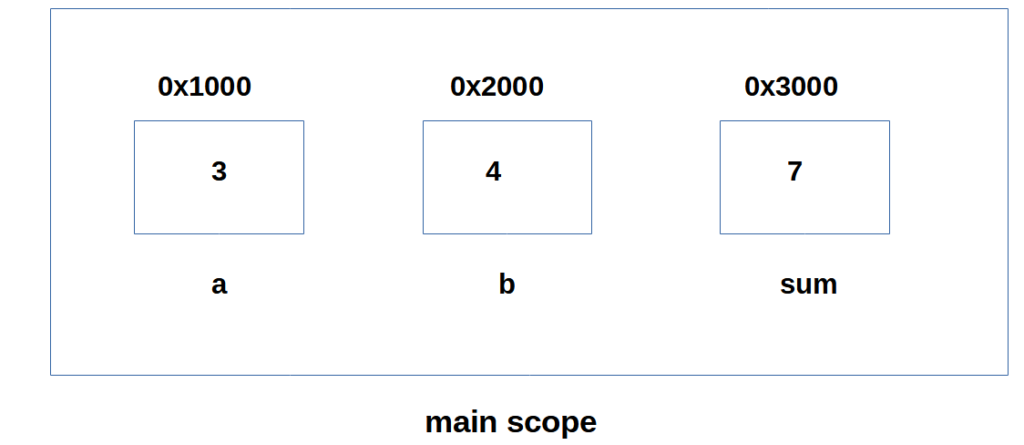
As the function ends, (main here) all the variables are erased from the memory and the scope from the memory deactivates.
