⯈Algorithm and its Characteristics
⯈Elementary Problems
- Addition of two numbers
- Calculate area and circumference of circle
- Calculate area of triangle
- Calculate simple interest
- Calculate slope and distance between two points
- Convert length in feet to inches
- Weighted score in exam
- Convert temperature in degree Celsius to Fahrenheit
- Swap two numbers
- Swap two numbers without using extra variable
- Overview of C Programming Language
- Getting started with C Programming Language
- Keywords
- Identifiers
- Constants
- Operators
- Expression Evaluation
- Mathematical expression to C equivalent expressions
- Datatypes
- Variables
- Integer representation in C
- Character representation in C
- Type conversion in C
- sizeof operator
- Comments
- Mathematical Functions
- input output statements
- width specifiers in C
- structure of a C program
- header files
- Compilation process of a C program
- Types of initializations.
⯈Basic C Programs
- C program to add two numbers
- C program to find area and circumference of circle
- C program to swap two numbers
- C program to swap two numbers without using extra variable
- C program to swap two numbers using bitwise XOR
- C program to convert temperature in Celsius to Fahrenheit
- C program to calculate gross salary of an employee
- C program to count number of digits in a positive integer
- C program to count number of digits in binary representation
- C program to count number of digits in base ‘K’
- C program to convert kilometer to meter, feet, inches and centimeters
- C program to find first and last digit of a number
- C program to find minimum number of currency denominations
- C program to convert cartesian coordinates to polar coordinates
- C program to find distance between two places on earth in nautical miles
- C program to find slope and distance between two points
- C program to add 1 to the number using ‘+’ operator
- C program to find maximum of two numbers using ternary operator
- C program to find maximum of three numbers using ternary operator
- C program to find kth bit of a number
- C program to find last four bits of a byte
- C Program to reset right most set bit of a number
C Program to find area and circumference of circle
We start the program by reading the necessary inputs and then conclude the program with calculating the outputs.
Input: radius (double)
Output: area (double) and circumference (double)
Reading the inputs,
Let ‘r’ be the radius.
scanf("%lf", &r);
No variable should be used unless it is declared, and since we have used scanf function we need stdio.h
#include<stdio.h>
int main()
{
double r;
scanf("%lf",&r);
...
}
It is good to give a user interface before we read the input, therefore, we write,
#include<stdio.h>
int main()
{
double r;
printf("Enter radius r:");
scanf("%lf", &r);
......
}
Calculating the output,
area = 3.142 * r * r;
cir = 2 * 3.142 * r;
No variables should be used unless they are declared. We go up the ladder and declare area and cir.
#include<stdio.h>
int main()
{
double r;
double area, cir;
printf("Enter radius:");
scanf("%lf",&r);
area = 3.142 * r * r;
cir = 2 * 3.142 * r;
.......
}
After we calculate all the outputs, its the time to display them.
Therefore, the final program becomes.
#include<stdio.h>
int main()
{
double r;
double area, cir;
printf("Enter radius:");
scanf("%lf",&r);
area = 3.142 * r * r;
cir = 2 * 3.142 * r;
printf("area = %lf\n",area);
printf("circumference = %lf\n",cir);
return 0;
}
Output window of above code
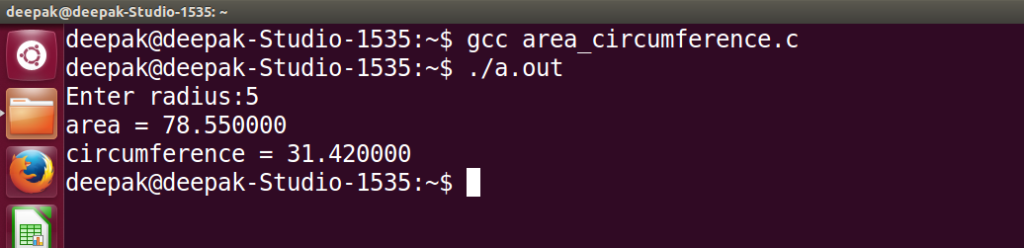
Memory diagram of above program
Scope after declaring all the variables,

Scope after reading the input (radius, r)
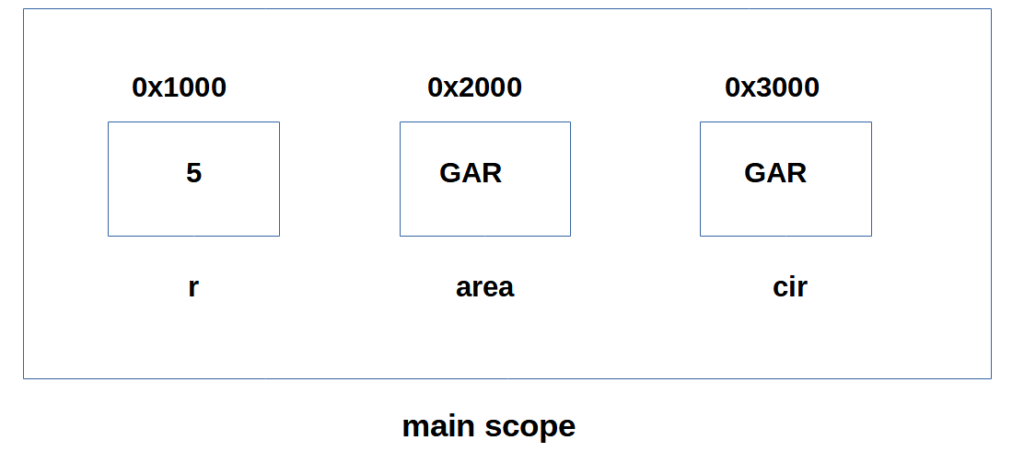
Scope after calculating the outputs,
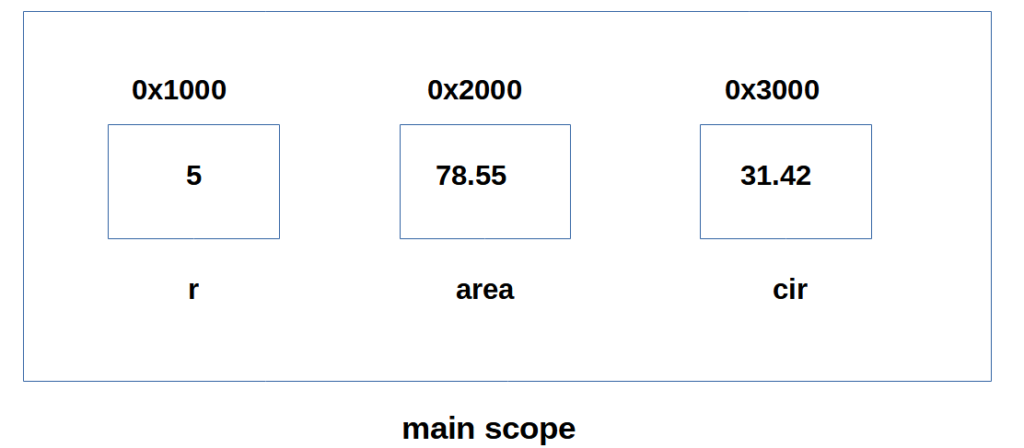
After the main program completes the scope deactivates
