Contents
Introduction to Problem Solving
⯈Algorithm and its Characteristics
⯈Elementary Problems
- Addition of two numbers
- Calculate area and circumference of circle
- Calculate area of triangle
- Calculate simple interest
- Calculate slope and distance between two points
- Convert length in feet to inches
- Weighted score in exam
- Convert temperature in degree Celsius to Fahrenheit
- Swap two numbers
- Swap two numbers without using extra variable
Basics of C Programming
- Overview of C Programming Language
- Getting started with C Programming Language
- Keywords
- Identifiers
- Constants
- Operators
- Expression Evaluation
- Mathematical expression to C equivalent expressions
- Datatypes
- Variables
- Integer representation in C
- Character representation in C
- Type conversion in C
- sizeof operator
- Comments
- Mathematical Functions
- input output statements
- width specifiers in C
- structure of a C program
- header files
- Compilation process of a C program
- Types of initializations.
⯈Basic C Programs
- C program to add two numbers
- C program to find area and circumference of circle
- C program to swap two numbers
- C program to swap two numbers without using extra variable
- C program to swap two numbers using bitwise XOR
- C program to convert temperature in Celsius to Fahrenheit
- C program to calculate gross salary of an employee
- C program to count number of digits in a positive integer
- C program to count number of digits in binary representation
- C program to count number of digits in base ‘K’
- C program to convert kilometer to meter, feet, inches and centimeters
- C program to find first and last digit of a number
- C program to find minimum number of currency denominations
- C program to convert cartesian coordinates to polar coordinates
- C program to find distance between two places on earth in nautical miles
- C program to find slope and distance between two points
- C program to add 1 to the number using ‘+’ operator
- C program to find maximum of two numbers using ternary operator
- C program to find maximum of three numbers using ternary operator
- C program to find kth bit of a number
- C program to find last four bits of a byte
- C Program to reset right most set bit of a number
C Program to swap two numbers
We start the programs, by reading the input. The input to the program is two numbers. Let the two numbers be ‘a’ and ‘b’ of type integer.
Input: ‘a’ and ‘b’ (int)
Output: Swapped values of ‘a’ and ‘b’
Reading input,
scanf("%d%d",&a,&b);
Let a = 5 and b = 7
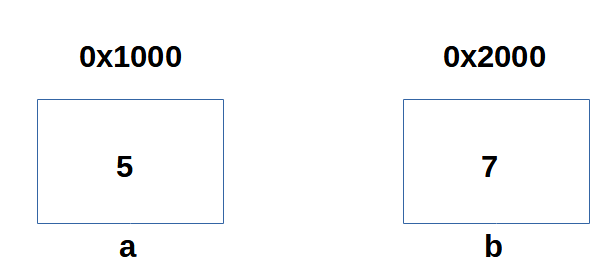
If we write, a = b, then the value of a will be over-written with ‘b’. A variable can store only one value at a given point of time.
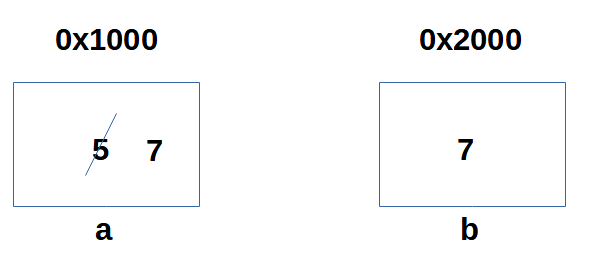
Since the value of ‘a’ is formatted with the value of ‘b’, before we format, we should store the value of ‘a’ in some variable.
So we write,
temp = a; (store a in temp, before we format)
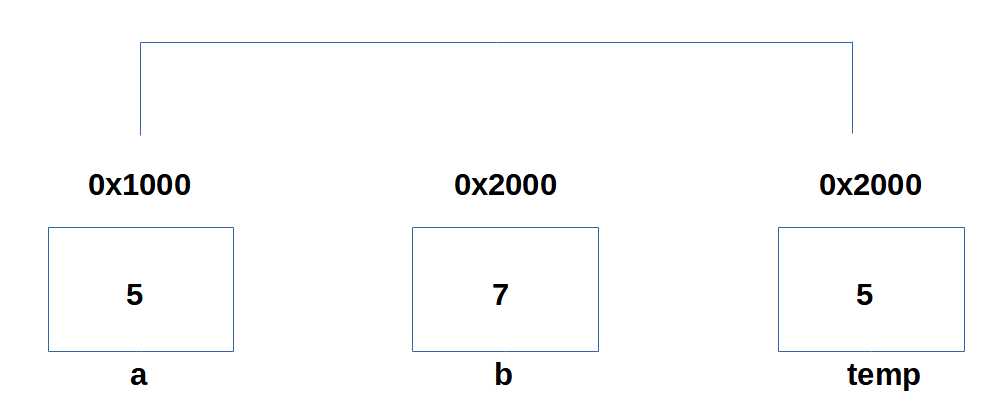
Now we can over-write a,
a = b
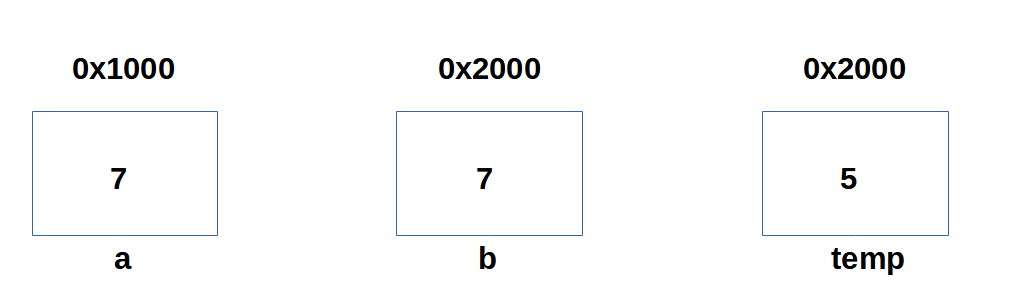
After we swap, the value of 'b' has to be 5, therefore,
temp = b

Logic
temp = a;
a = b;
b = temp;
C program
#include<stdio.h>
int main()
{
int a, b;
int temp;
printf("Enter two numbers:");
scanf("%d%d",&a,&b);
temp = a;
a = b;
b = temp;
printf("After swapping\n");
printf("a=%d\n",a);
printf("b= %d\n",b);
return 0;
}
Output window of above code
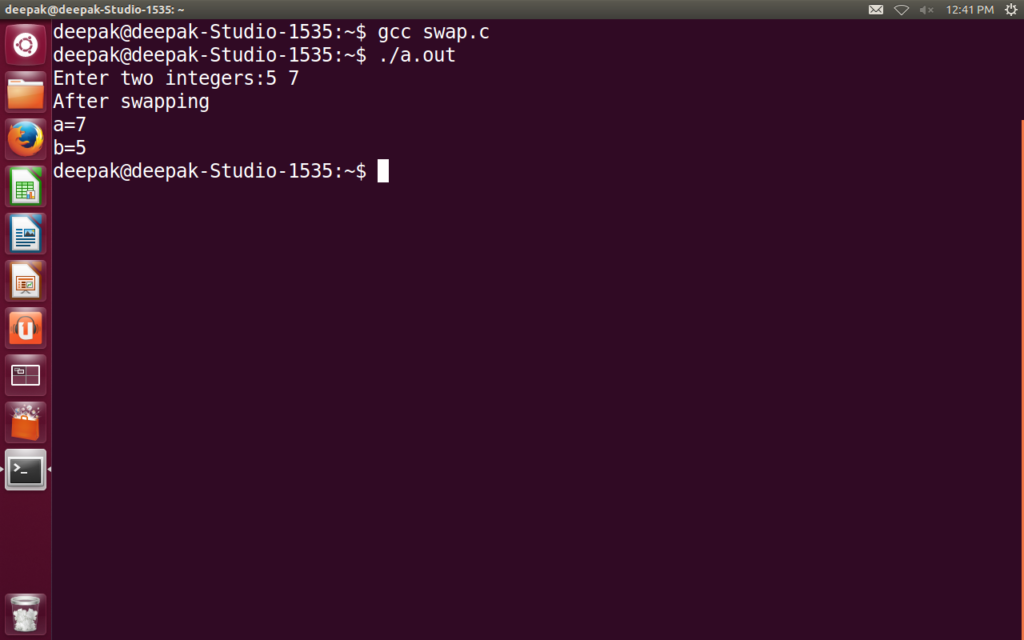