⯈Algorithm and its Characteristics
⯈Elementary Problems
- Addition of two numbers
- Calculate area and circumference of circle
- Calculate area of triangle
- Calculate simple interest
- Calculate slope and distance between two points
- Convert length in feet to inches
- Weighted score in exam
- Convert temperature in degree Celsius to Fahrenheit
- Swap two numbers
- Swap two numbers without using extra variable
- Overview of C Programming Language
- Getting started with C Programming Language
- Keywords
- Identifiers
- Constants
- Operators
- Expression Evaluation
- Mathematical expression to C equivalent expressions
- Datatypes
- Variables
- Integer representation in C
- Character representation in C
- Type conversion in C
- sizeof operator
- Comments
- Mathematical Functions
- input output statements
- width specifiers in C
- structure of a C program
- header files
- Compilation process of a C program
- Types of initializations.
⯈Basic C Programs
- C program to add two numbers
- C program to find area and circumference of circle
- C program to swap two numbers
- C program to swap two numbers without using extra variable
- C program to swap two numbers using bitwise XOR
- C program to convert temperature in Celsius to Fahrenheit
- C program to calculate gross salary of an employee
- C program to count number of digits in a positive integer
- C program to count number of digits in binary representation
- C program to count number of digits in base ‘K’
- C program to convert kilometer to meter, feet, inches and centimeters
- C program to find first and last digit of a number
- C program to find minimum number of currency denominations
- C program to convert cartesian coordinates to polar coordinates
- C program to find distance between two places on earth in nautical miles
- C program to find slope and distance between two points
- C program to add 1 to the number using ‘+’ operator
- C program to find maximum of two numbers using ternary operator
- C program to find maximum of three numbers using ternary operator
- C program to find kth bit of a number
- C program to find last four bits of a byte
- C Program to reset right most set bit of a number
C Program to swap two numbers using bitwise XOR
We start the programs, by reading the input. The input to the program is two numbers. Let the two numbers be ‘a’ and ‘b’ of type integer.
Input: ‘a’ and ‘b’ (int)
Output: Swapped values of ‘a’ and ‘b’
Reading input,
scanf("%d%d",&a,&b);
Let a = 5 and b = 7
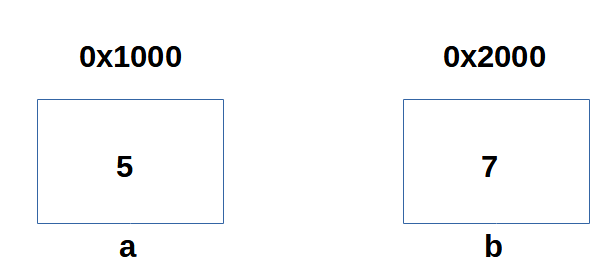
Bit pattern of a and b
Please refer to this on how to get the bit pattern.
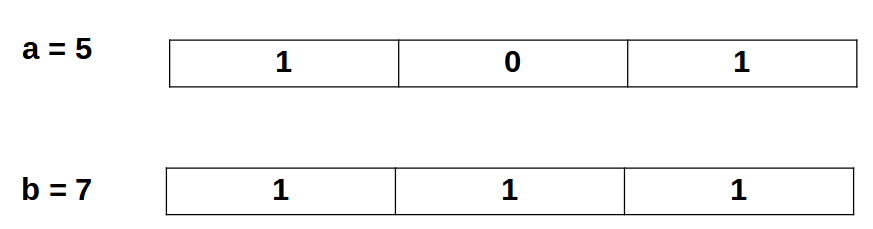
We perform the XOR of a and b (a ^ b) and we get the answer as 2. This answer we store either in a or b.
Note: XOR forms an abelian group under set of integers.
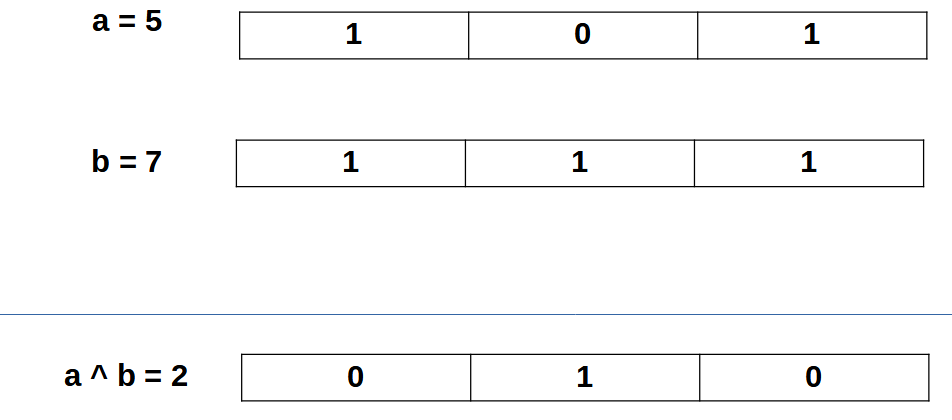
If we store the answer of a^b in a, that is,
a = a ^ b;
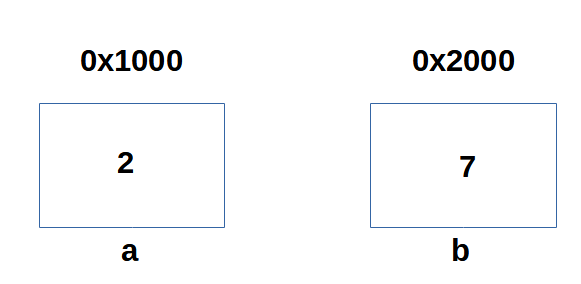
If we perform a^b again, we get 5. We want to store the 5 in b, therefore we write,
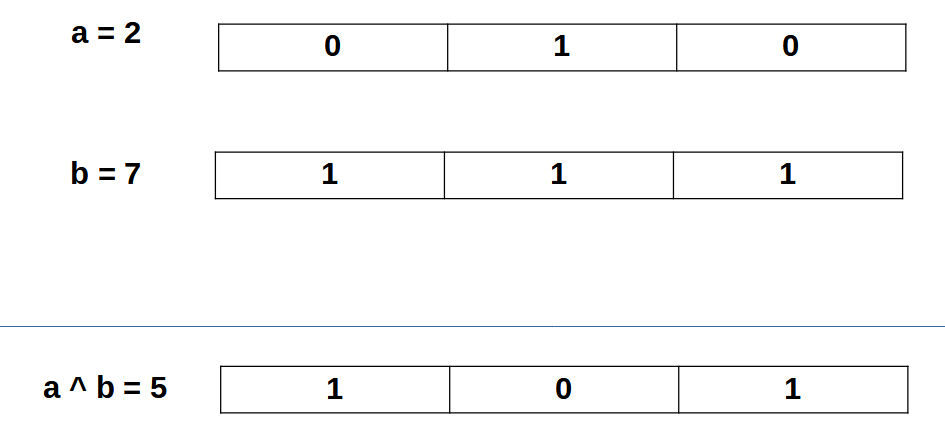
b = a ^ b;
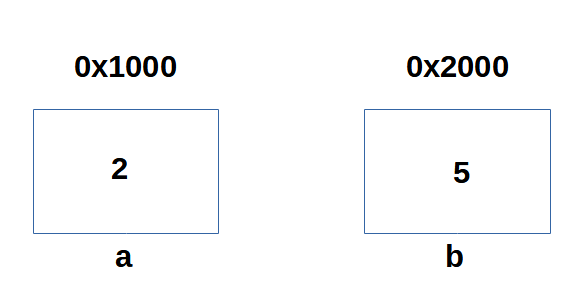
To get 7 back, we write a^b
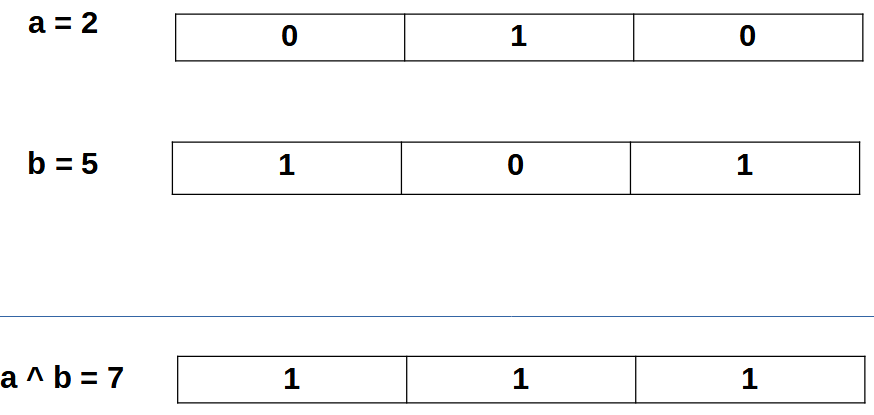
a = a ^ b;
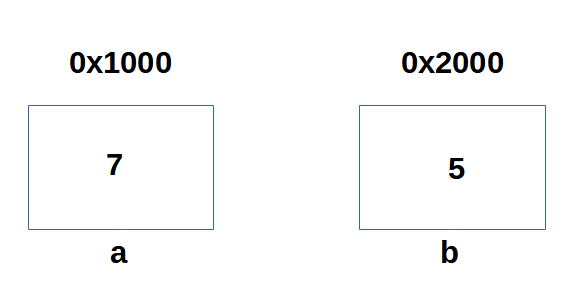
C Program
#include<stdio.h>
int main()
{
int a, b;
printf("Enter two numbers:");
scanf("%d%d",&a,&b);
a = a ^ b;
b = a ^ b;
a = a ^ b;
printf("a=%d\nb=%d\n",a,b);
return 0;
}
Note: This logic can only be applied on integers as bitwise operators are only applicable on integers.
Output window
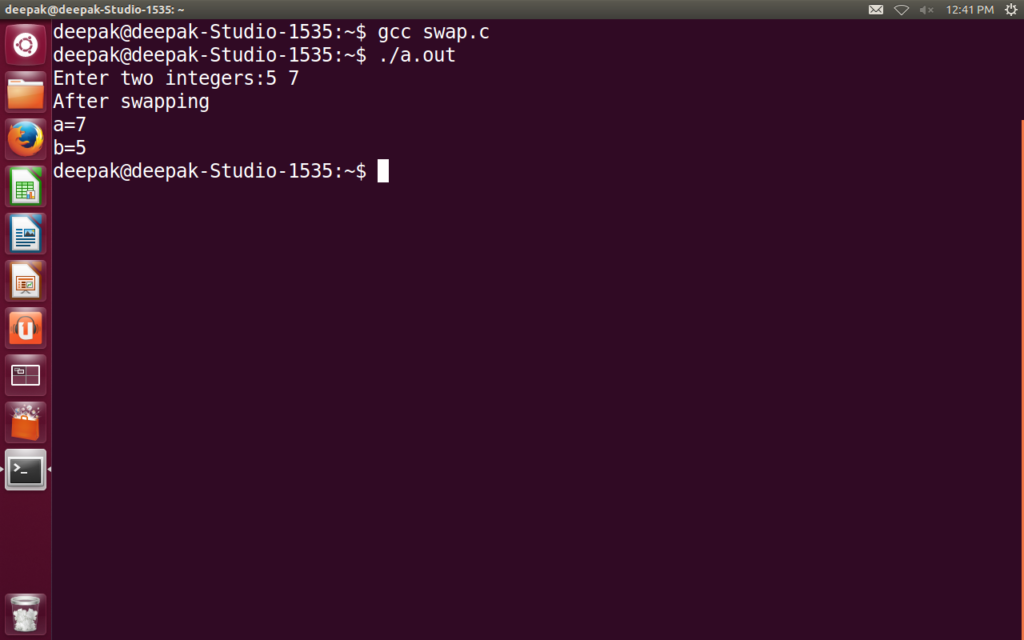