⯈Algorithm and its Characteristics
⯈Elementary Problems
- Addition of two numbers
- Calculate area and circumference of circle
- Calculate area of triangle
- Calculate simple interest
- Calculate slope and distance between two points
- Convert length in feet to inches
- Weighted score in exam
- Convert temperature in degree Celsius to Fahrenheit
- Swap two numbers
- Swap two numbers without using extra variable
- Overview of C Programming Language
- Getting started with C Programming Language
- Keywords
- Identifiers
- Constants
- Operators
- Expression Evaluation
- Mathematical expression to C equivalent expressions
- Datatypes
- Variables
- Integer representation in C
- Character representation in C
- Type conversion in C
- sizeof operator
- Comments
- Mathematical Functions
- input output statements
- width specifiers in C
- structure of a C program
- header files
- Compilation process of a C program
- Types of initializations.
⯈Basic C Programs
- C program to add two numbers
- C program to find area and circumference of circle
- C program to swap two numbers
- C program to swap two numbers without using extra variable
- C program to swap two numbers using bitwise XOR
- C program to convert temperature in Celsius to Fahrenheit
- C program to calculate gross salary of an employee
- C program to count number of digits in a positive integer
- C program to count number of digits in binary representation
- C program to count number of digits in base ‘K’
- C program to convert kilometer to meter, feet, inches and centimeters
- C program to find first and last digit of a number
- C program to find minimum number of currency denominations
- C program to convert cartesian coordinates to polar coordinates
- C program to find distance between two places on earth in nautical miles
- C program to find slope and distance between two points
- C program to add 1 to the number using ‘+’ operator
- C program to find maximum of two numbers using ternary operator
- C program to find maximum of three numbers using ternary operator
- C program to find kth bit of a number
- C program to find last four bits of a byte
- C Program to reset right most set bit of a number
Logical operators in C
Logical operators give the answer in the form of true or false only. These operators are used to connect one or more conditions. There are three logical operators in C.
You may refer the video as posted at the end.
Logical NOT(!)
- It is a unary operator.
- The logical NOT (!) operator gives answer as true, if the input is false, and the answer as false if the input is true.
Truth Table:
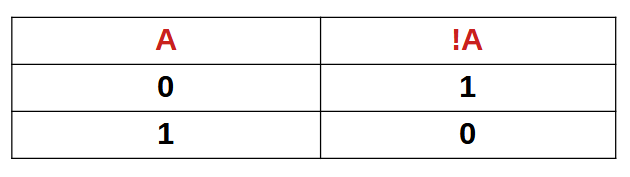
Examples:
- !1 = 0
- !0 = 1
- !-1 = 0 (since -1 is true)
- !19 = 0 (since 19 is true)
- !’A’ = 0 (Since ‘A’ is true)
We have already discussed about truth and falsity values in C here.
Logical AND (&&)
- It is a binary operator.
- This operator gives the answer as true if both the operands are true, else the output is false.
Truth Table
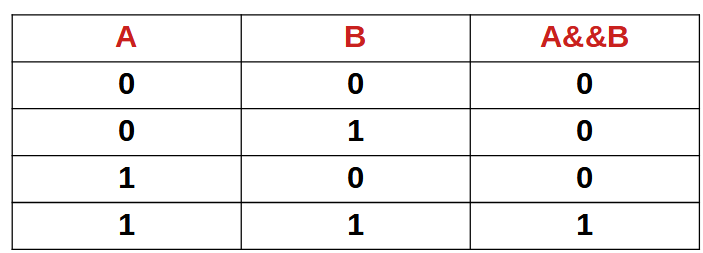
Examples:
- 23 && -4 = 1, (since 23 is True and -4 is also True)
- ‘A’ && 21 = 1, (since ‘A’ is True and 21 is also True)
- 9 && 0 = 0
We have already discussed about truth and falsity values in C here.
Short circuiting in Logical AND
If the first operand in logical AND is false, the compiler doesn’t evaluate the second operator, because FALSE && Anything = FALSE. This is called as short circuiting in logical AND.
Example:
Let a = 0 and b = 4
c = a && ++b,
The values of
a = 0, b = 4 and c = 0
Note: the expression ++b won’t be evaluated as the first operand in Logical AND is false.
Logical OR (||)
- It is a binary operator.
- This operator gives the answer as true if anyone of the operands is true, else the output is false.
Truth Table
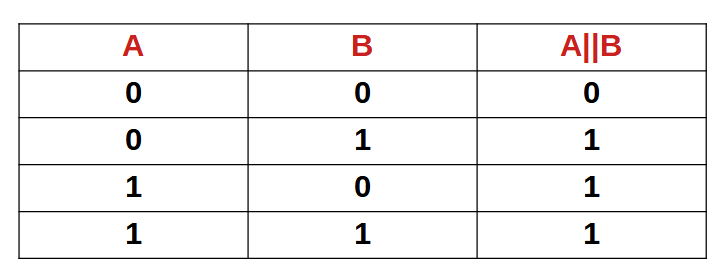
Examples:
- 23 || -4 = 1, (since 23 is True and -4 is also True)
- ‘A’ || 21 = 1, (since ‘A’ is True and 21 is also True)
- 9 || 0 = 1
- 0||0 = 0
We have already discussed about truth and falsity values in C here.
Short circuiting in Logical OR
If the first operand in logical OR is true, the compiler doesn’t evaluate the second operator, because TRUE && Anything = TRUE. This is called as short circuiting in logical OR.
Example:
Let a = 1 and b = 4
c = a || ++b,
The values of
a = 1, b = 4 and c = 1
Note: the expression ++b won’t be evaluated as the first operand in Logical OR is true.