⯈Algorithm and its Characteristics
⯈Elementary Problems
- Addition of two numbers
- Calculate area and circumference of circle
- Calculate area of triangle
- Calculate simple interest
- Calculate slope and distance between two points
- Convert length in feet to inches
- Weighted score in exam
- Convert temperature in degree Celsius to Fahrenheit
- Swap two numbers
- Swap two numbers without using extra variable
- Overview of C Programming Language
- Getting started with C Programming Language
- Keywords
- Identifiers
- Constants
- Operators
- Expression Evaluation
- Mathematical expression to C equivalent expressions
- Datatypes
- Variables
- Integer representation in C
- Character representation in C
- Type conversion in C
- sizeof operator
- Comments
- Mathematical Functions
- input output statements
- width specifiers in C
- structure of a C program
- header files
- Compilation process of a C program
- Types of initializations.
⯈Basic C Programs
- C program to add two numbers
- C program to find area and circumference of circle
- C program to swap two numbers
- C program to swap two numbers without using extra variable
- C program to swap two numbers using bitwise XOR
- C program to convert temperature in Celsius to Fahrenheit
- C program to calculate gross salary of an employee
- C program to count number of digits in a positive integer
- C program to count number of digits in binary representation
- C program to count number of digits in base ‘K’
- C program to convert kilometer to meter, feet, inches and centimeters
- C program to find first and last digit of a number
- C program to find minimum number of currency denominations
- C program to convert cartesian coordinates to polar coordinates
- C program to find distance between two places on earth in nautical miles
- C program to find slope and distance between two points
- C program to add 1 to the number using ‘+’ operator
- C program to find maximum of two numbers using ternary operator
- C program to find maximum of three numbers using ternary operator
- C program to find kth bit of a number
- C program to find last four bits of a byte
- C Program to reset right most set bit of a number
Type conversion in C
Type conversion says converting one datatype to another. There will be some situations in programming where we would want to convert from one datatype to another. It is also called as type casting.
Say for example, we want to calculate 2/3, which is 0.67 mathematically and 0 programmatically, so we would want either 2 or 3 to convert to double number.
There are two types of “type conversion”
You may watch the video posted at the end of this page.
Implicit type conversion
The implicit type conversion is automatically done by the compiler.
Example 1:
int a = 2.3;
we are trying to store double number in integer, which is not possible, and the compiler converts this to an integer value by truncating the decimal point.
Therefore, a = 2;
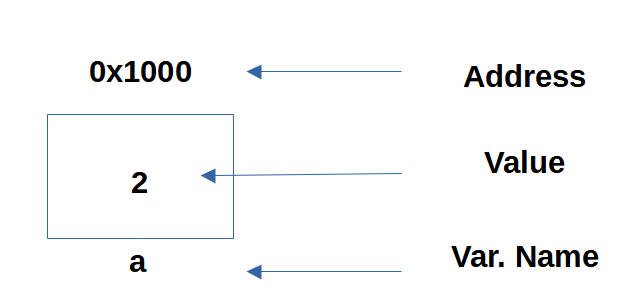
Example 2:
int y = ‘A’;
We see that the RHS is character and the LHS is an integer. The character will be converted to an integer and the ASCII value of ‘A’, which is 65, will be stored. Therefore, y = 65
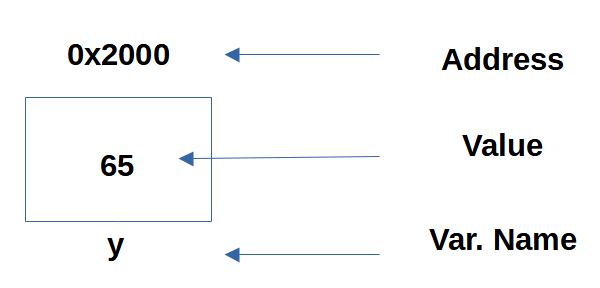
Example 3:
double m = 3;
We see that the RHS value is integer, and the LHS is double, integer is upgraded to double and 3.0 will be stored in m.
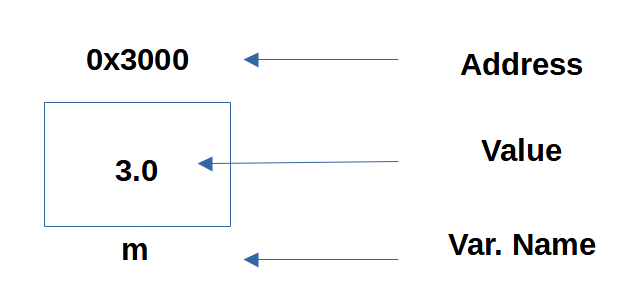
Explicit type conversion
The explicit type conversion is the forceful conversion done by the programmer. It is not done by the compiler.
General Syntax:
variable=(data_type)variable_name or value;
Example 1:
int a = 2;
int b = 3;
double c = a/b; (The value of var, c, will be 0, since int/int = int)
To get the value, 0.67, we need to convert either ‘a’ or ‘b’ or both to double. But there might be situations where we don’t want to change the datatype of the variables. That is where the explicit type conversion comes into play.
Explicit type conversion is converting one datatype to another datatype momentarily.
Therefore, we write
double d = (double)a/b;
The value of d will be 0.67.
The variable ‘a’ will be converted to double only where it is written as (double)a, rest at all the places the datatype of ‘a’ will be an integer.