⯈Algorithm and its Characteristics
⯈Elementary Problems
- Addition of two numbers
- Calculate area and circumference of circle
- Calculate area of triangle
- Calculate simple interest
- Calculate slope and distance between two points
- Convert length in feet to inches
- Weighted score in exam
- Convert temperature in degree Celsius to Fahrenheit
- Swap two numbers
- Swap two numbers without using extra variable
- Overview of C Programming Language
- Getting started with C Programming Language
- Keywords
- Identifiers
- Constants
- Operators
- Expression Evaluation
- Mathematical expression to C equivalent expressions
- Datatypes
- Variables
- Integer representation in C
- Character representation in C
- Type conversion in C
- sizeof operator
- Comments
- Mathematical Functions
- input output statements
- width specifiers in C
- structure of a C program
- header files
- Compilation process of a C program
- Types of initializations.
⯈Basic C Programs
- C program to add two numbers
- C program to find area and circumference of circle
- C program to swap two numbers
- C program to swap two numbers without using extra variable
- C program to swap two numbers using bitwise XOR
- C program to convert temperature in Celsius to Fahrenheit
- C program to calculate gross salary of an employee
- C program to count number of digits in a positive integer
- C program to count number of digits in binary representation
- C program to count number of digits in base ‘K’
- C program to convert kilometer to meter, feet, inches and centimeters
- C program to find first and last digit of a number
- C program to find minimum number of currency denominations
- C program to convert cartesian coordinates to polar coordinates
- C program to find distance between two places on earth in nautical miles
- C program to find slope and distance between two points
- C program to add 1 to the number using ‘+’ operator
- C program to find maximum of two numbers using ternary operator
- C program to find maximum of three numbers using ternary operator
- C program to find kth bit of a number
- C program to find last four bits of a byte
- C Program to reset right most set bit of a number
Width specifiers
- Width specifiers says what is the maximum number of digits or characters that we want to read or print.
- This we can use both with the scanf and printf.
- If used with scanf, we can limit the number of characters or digits that we can read.
- If used with printf, we can limit the number of characters or numbers that we can print.
Examples
Example 1:
int a;
If we want to read only a two-digit number from the scanf statement, we would write,
scanf(“%2d”,&a);
Even if we enter more than two digits the starting two digits (two characters, if we consider each number as a character, even ‘-‘ sign will be character) will be taken as the input and rest all the digits are discarded.
#include<stdio.h>
int main()
{
int a;
printf("Enter number:");
scanf("%2d",&a);
printf("a = %d\n",a);
return 0;
}
Output Window of above code
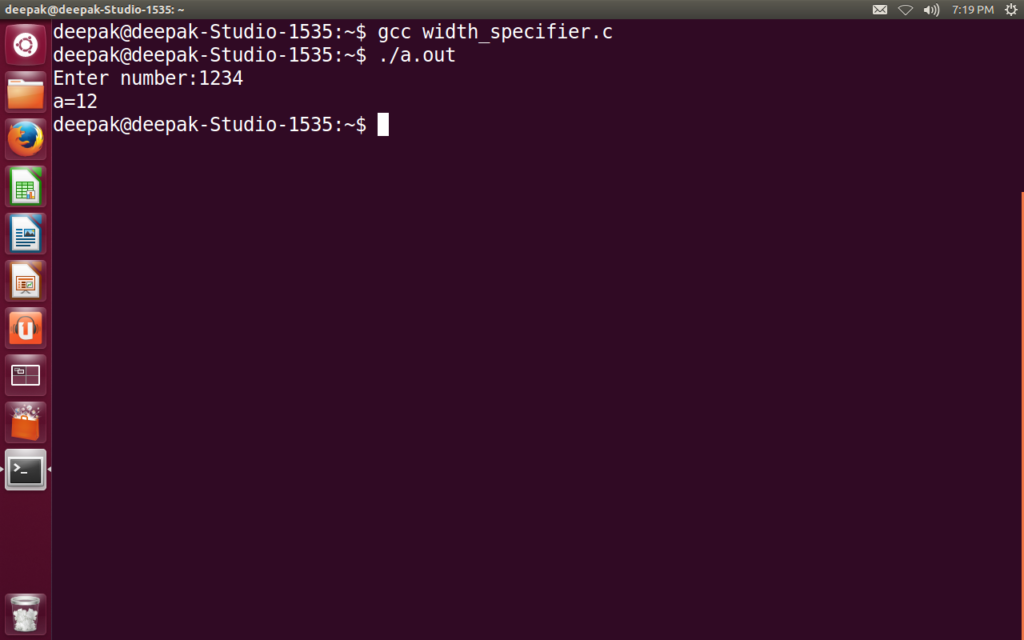
We can clearly see that, if we enter number as 1234, only the starting two digits are stored in ‘a’ and the rest are discarded.
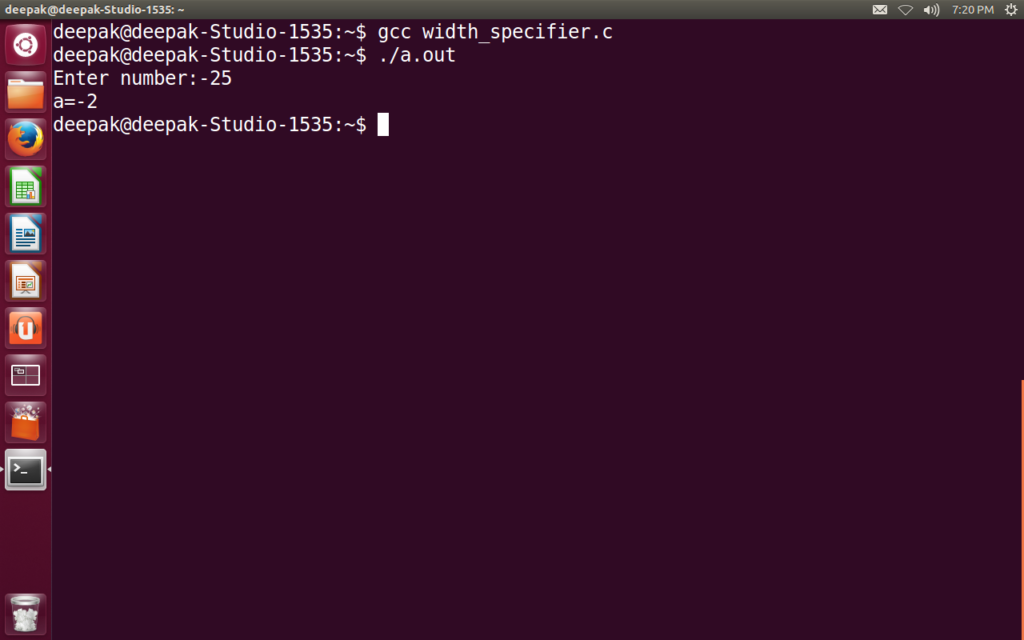
If we enter number as -25, only the starting two characters -2 (- and 2) are stored and rest all are discarded.
Example 2:
If we want to print the number of digits after the decimal point in case of double / float number, we can use width specifiers. The decimal number will be rounded-off to nearest place.
double a = 9.2489;
If we want to print the value of ‘a’, up to two decimal places, we will write
printf(“%.2lf“,a);, It prints, 9.25
If you want to print up to three digits, we will wite,
printf(“%.3lf“,a);, It prints 9.249
#include<stdio.h>
int main()
{
double a = 9.2489;
printf("%.2lf\n",a);
printf("%.3lf\n",a);
return 0;
}
Output window of above code
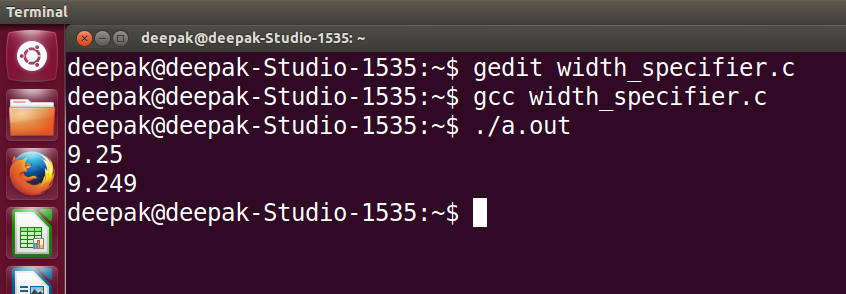