⯈Algorithm and its Characteristics
⯈Elementary Problems
- Addition of two numbers
- Calculate area and circumference of circle
- Calculate area of triangle
- Calculate simple interest
- Calculate slope and distance between two points
- Convert length in feet to inches
- Weighted score in exam
- Convert temperature in degree Celsius to Fahrenheit
- Swap two numbers
- Swap two numbers without using extra variable
- Overview of C Programming Language
- Getting started with C Programming Language
- Keywords
- Identifiers
- Constants
- Operators
- Expression Evaluation
- Mathematical expression to C equivalent expressions
- Datatypes
- Variables
- Integer representation in C
- Character representation in C
- Type conversion in C
- sizeof operator
- Comments
- Mathematical Functions
- input output statements
- width specifiers in C
- structure of a C program
- header files
- Compilation process of a C program
- Types of initializations.
⯈Basic C Programs
- C program to add two numbers
- C program to find area and circumference of circle
- C program to swap two numbers
- C program to swap two numbers without using extra variable
- C program to swap two numbers using bitwise XOR
- C program to convert temperature in Celsius to Fahrenheit
- C program to calculate gross salary of an employee
- C program to count number of digits in a positive integer
- C program to count number of digits in binary representation
- C program to count number of digits in base ‘K’
- C program to convert kilometer to meter, feet, inches and centimeters
- C program to find first and last digit of a number
- C program to find minimum number of currency denominations
- C program to convert cartesian coordinates to polar coordinates
- C program to find distance between two places on earth in nautical miles
- C program to find slope and distance between two points
- C program to add 1 to the number using ‘+’ operator
- C program to find maximum of two numbers using ternary operator
- C program to find maximum of three numbers using ternary operator
- C program to find kth bit of a number
- C program to find last four bits of a byte
Constants in C
A constant is something whose value doesn’t change during the execution of the program.
There are two types of constants at the highest level. They are:
Types of constants in C
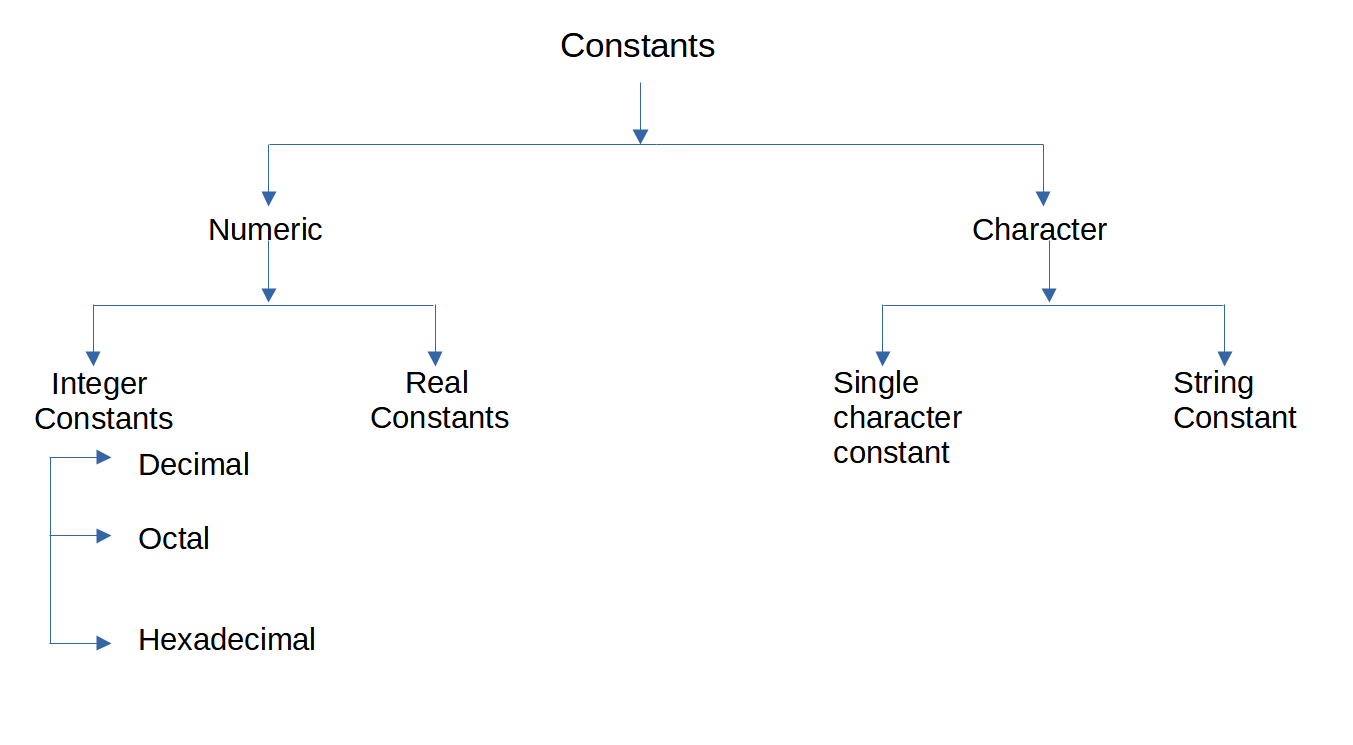
Numeric Constants
These constants contain only numeric values. There are two types of numeric constants, they are:
Integer constants
Integer constants are the one which don’t contain the decimal point.
Ex: 2, +3, -4, etc.
There are three types of integer constants.
We will discuss each of these in the coming sections.
Decimal integer constants
Decimal integer constants are the numbers which have base – 10. Numbers from 0 to 9 can be used to form any number. The number may be positive or negative.
Ex: +123, -89, 45
Octal integer constants
Octal integer constants are the numbers which have base – 8 system, it means we can use number from 0 to 7. The numbers may be positive or negative.
If we write the number, 45, the number can be both decimal as well as octal. This is ambiguity. To resolve this, octal numbers begin with 0.
Therefore 45 is decimal, whereas 045 is octal.
Ex: 022, -017
Note: 0 is octal in C programming language but is decimal in Java.
The following are invalid octal constants.
- 018 (contains 8, octal numbers are formed from 0 – 7 only)
- 15 (don’t start with 0)
Hexa decimal integer constants
Hexa decimal constants have the base 16. 16 different values can be used. It uses the numbers from 0-9 and A-F. Hexa-decimal numbers may be positive or negative.
A – 10 , B – 11, C – 12, D-13, E-14, F-15
The alphabets A-F can either be written in upper case or lower case.
If we write the number 45, this number can be decimal or hexa-decimal. This is case of ambiguity. To overcome this hexa-decimal numbers, begin with 0x.
Therefore, 45 is decimal whereas 0x45 is hexa-decimal.
Ex: 0x00, 0x12EA, 0x45, -0x46
Real Constants
Real constants are one which have decimal point. Real constants can be positive or negative.
Ex: 3.14, 9.24, -8.4, 2.0
Note:
- 2 is integer, whereas 2.0 is real constant.
- we can write 2.0 as 2. (note that after decimal point, we didn’t write anything)
Real constants can also be written in scientific notation.
Ex:
- 123.45 in scientific notation in mathematics is written as, 1.2345*10². In C programming we write it as, 1.2345E2. Here E represents 10 to the power.
- 0.0034 in scientific notation in mathematics is written as, 3.4*10-3 . In C programming we write it as, 3.4E-3. Here E represents 10 to the power.
- -53.5 can be written as as -5.3E1
Character constants
Character constants are made up of alphabets, digits and special characters. There are two types of character constants.
Single character constants
Anything written in single quotes and is of single in length is called as single character constants.
Ex: ‘a’, ‘Q’, ‘4’, ‘%’
The following are not single characters constants.
- a (not written in single quotations)
- ‘ab’ (Even though written in single quotes, but is not of single in length)
String constants
Anything written in double quotes becomes a string. The formal definition of string is an array of characters terminated with NULL.
Ex: “BHARAT”, “INDIA”, “123”, “A1”, “123@abc”
To Summarize
If we write,
4 — It is an integer constant.
4 .0 — It is a real constant. (has decimal point)
‘4’ — It is a single character constant. (written in single quote)
“4” — It is a string constant.